Guide
What is a REST API?
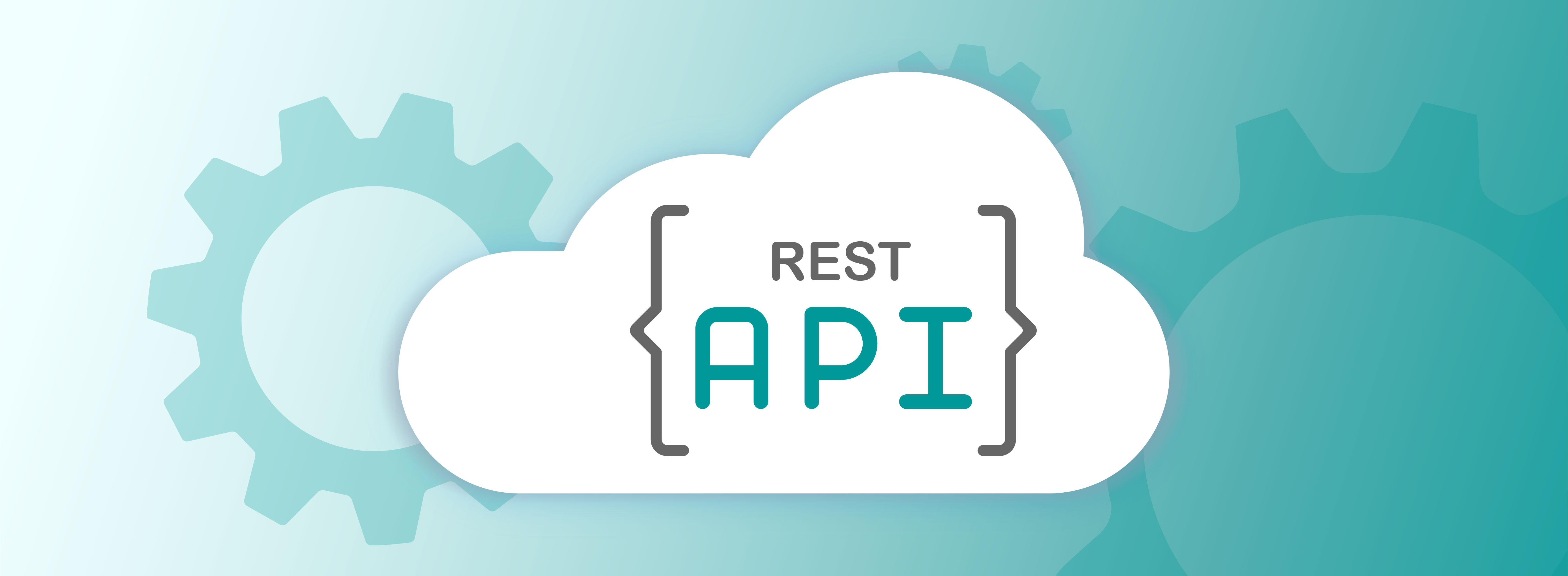
Rest API relies on the client-server paradigm: the client is triggering the data exchange by posing a request, the server processes the request and concludes the data exchange by sending a response.
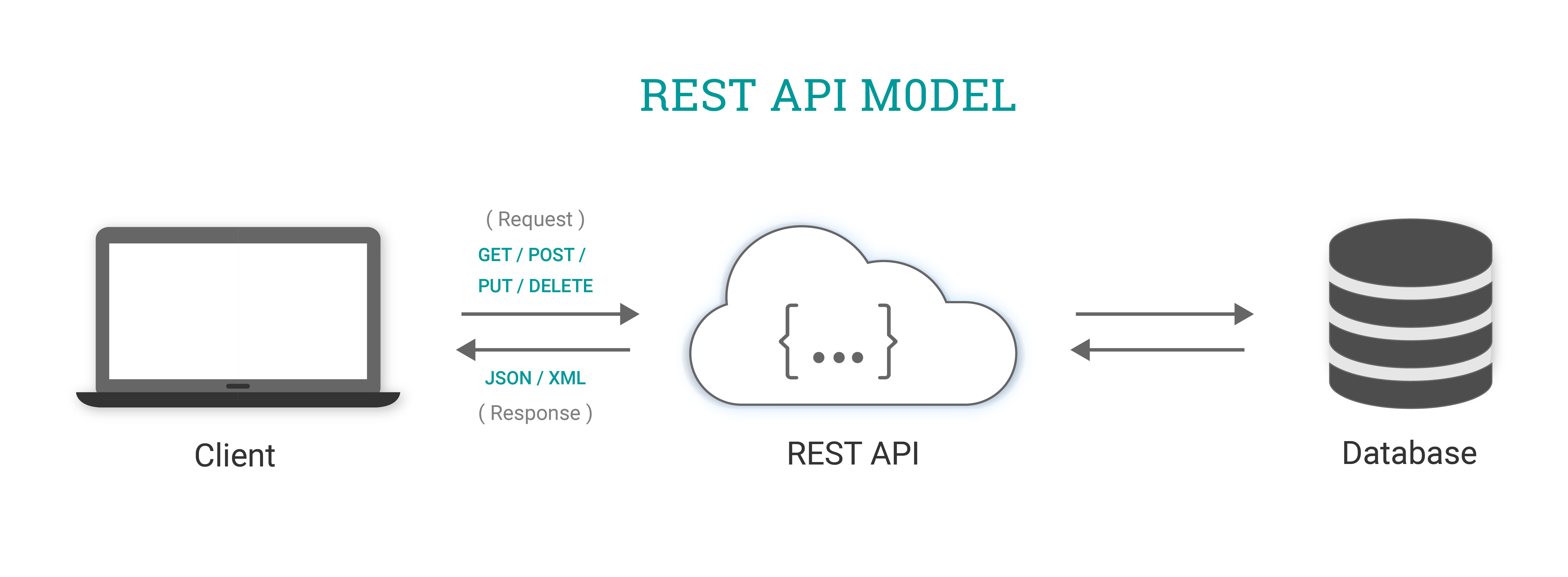
Before we tackle the inner workings of a REST API further, some terms shall be coined:
A few words on terminology
Clients:
It may be a person or program using the API. The client makes requests to the API to retrieve some information or change something within the application.
For example, a web page (web app) in a web browser can act as a client; it interacts with the webserver’s API to retrieve content from it, renders it into a new page and your browser displays this page on your screen.
Resources:
Resources are information that the API can provide to the client. As an example, a resource in SICON.OS API could be an app, a device, an event, a user and so on. Each resource encompasses a unique identifier, called the uniform resource identifier or URL.
URLs include the network location of the resource and protocol to access it. So, URLs can be used to directly access a resource by a client, that understands the protocol, e.g., a browser for HTTP URLs.
Server:
Server are employed by the application that receives client requests and contain resources that the client wants to have access to.
REST:
REST stands for Representational State Transfer. This means that based upon the client's request for a particular resource using a REST API, the server sends response back in form of standardized representation of the actual state of the resource.
REST API:
(Also called a RESTful API) is a set of guidelines that software can use to communicate over the internet to make integrations simple and scalable.
HTTP and REST API methods
RESTful API best practices comprise of four essential methods. These methods (operations) are labelled CRUD methods. CRUD is short for CREATE, READ, UPDATE, DELETE.
REST relies heavily on HTTP. Each above mentioned operation uses a corresponding HTTP method:
POST ≙ create
GET≙ read
PUT ≙ update (modification)
DELETE ≙ delete
HTTP status codes
Each HTTP response come with an HTTP status code. They are divided into five subsets. The first number indicates to which of them a status code belongs – like “200 OK” or the infamous “404 Not Found”:
1xx - informational
2xx - success
3xx - redirection
4xx - client error
5xx - server error
Here is a screenshot of an actual HTTP request as an example:
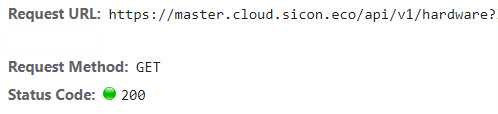
Security aspects:
Authenticity
To protect the resources from illegitimate access, a REST API can require authentication for a subset of requests. So, a client has to authenticate (e.g., via a JWT token or via username and password) towards the server to let the server know, who is sending the request. This information can be stored in session cookies.
Authority
A server can define privileges and can bundle them to roles. During the processing of the request, the privileges of the client’s user are looked up to determine if it is authorized to compute a positive response.
Confidentiality
To protect the data transferred between server and client, the connection is usually encrypted, so that eavesdropper cannot read out resource data, or credentials and cannot issue requests posing as the legitimate user (replay attack, man-in-the-middle attack).
Rest API workflow
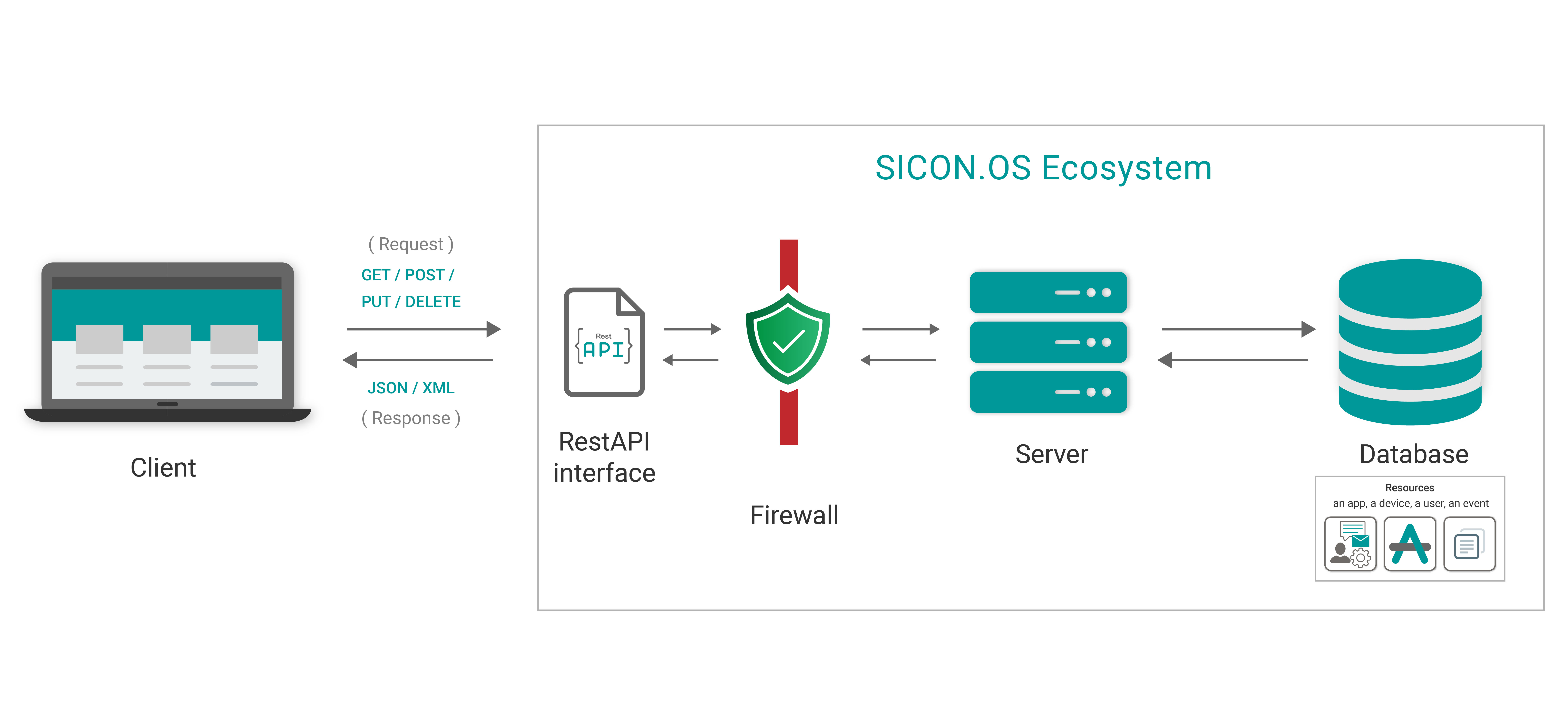
An HTTP client, e.g., a browser is sending a request to the server. In case of a resource request that requires authorization, the server checks if there is already a valid authorization attached to the session (server-side cookie). If not an error response (“Authorization failed”/” Unauthorized”) is sent to the client along with the HTTP Code 401. If a valid authorization does exist, the server uses the request parameters to compute a response. Depending on the outcome of the response computation (success or error) a matching http status code and if applicable a payload is sent back to the client. The client app can now process the response and generate output for the user, e.g., a page or a dialog.
SICON.OS REST API Examples
GET method - receiving data in a convenient format
All resources in REST are entities. They can be independent like:
GET /devices - get all devices

GET /devices/456 - get a device with id = 456

GET /events - get all events

There are also entities which are dependent and are derived from their parent models:
GET /devices/456/update-available- get all the updates-available that a device with id = 456 has

The above examples exhibit that GET means getting the entity you request. When performing the same request again, the identical data is received, or we can say it is idempotent. A successful request returns a combination of entity representation and status code 200 (OK). If there is an error you will get back code 404 (Not Found), 400 (Bad Request) or 5xx (Server Error).
Screenshot:
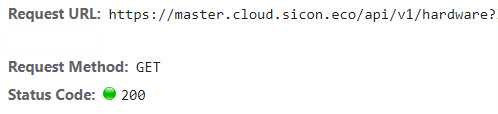
POST method – to create a new entity
POST /users
When creating a new entity, you send JSON in the request body.
For example:
{
"Firstname": "John",
"Lastname": "Eva",
"Email": "test@dummy.com",
"User": "john.eva",
"Password": "MySecret"
}
If same data is sent again as a new POST request, then it will lead to creation of an duplicate entity but with a different identifier as POST request is non-idempotent.
After that you will get the result which may be status code 200 (OK), for example. Then the response will contain the data of the saved entity.
For example:
{
"ID": 15,
"Firstname": "John",
"Lastname": "Eva",
"Email": "test@dummy.com",
"User": "john.eva",
"System": false
}
Response with status code 201 (created) can also be returned by the server, resulting in the creation of a new entity.
PUT method - modifying an existing entry
It is used to update entities. The request requires a means to identify the entity. The request body shall contain updated entity data it refers to.
PUT /devices/456 - update a device entity with id = 456

The changes should be sent as parameters with the request. If updated successfully the request returns code 200 (OK) and the representation of the updated entity.
DELETE method - removing an existing entry
The request only requires data to identify the entity, e.g., an id or a unique set of properties, if the call supports that.
DELETE /users/123 - delete the user with id = 123
Status code 200 (OK) is returned as a response by the server once the entity removal is successful with body containing data about the status of entity. DELETE can be considered as an idempotent request. It can also return code 204 (No Content) without the response body.
Reissuing the same request for the same entity should return an error response with the http status 404 (Not Found) because that resource is already removed and no longer accessible.